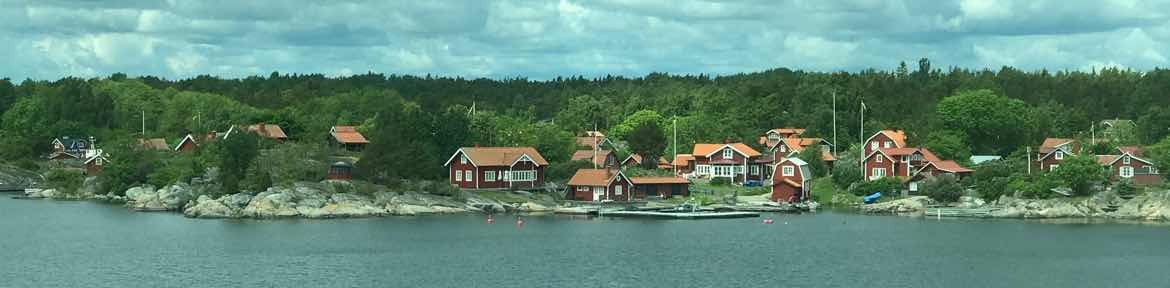
Technipelago Blog Stuff that we learned...
Custom error response for Spring REST endpoints
It's easy to customize the error response from REST endpoints in Spring Boot. This blog post explains how to add a custom error code to the response.
The default error response from a Spring Boot REST endpoint looks something like this:
{ "error": "Not Found", "exception": "com.mycompany.rest.MyException", "message": "A record with value [foo] was not found", "path": "/foo", "status": 404, "timestamp": 1507117452642 }
If we want to include custom information into the error response it's easy. Just add an ErrorAttributes bean to the spring context.
The following code snippet checks if the thrown exception is a custom exception MyException. This exception type contains a code attribute that holds a unique error code. We want to include that code as a specific key in the error response.
@SpringBootApplication @EnableResourceServer public class MyApplication { public static void main(String[] args) { SpringApplication.run(MyApplication.class, args); } @Bean public ErrorAttributes errorAttributes() { return new DefaultErrorAttributes() { @Override public MapgetErrorAttributes(RequestAttributes requestAttributes, boolean includeStackTrace) { final Map<String, Object> errorAttributes = super.getErrorAttributes(requestAttributes, includeStackTrace); // Customize the default entries in errorAttributes by adding the error code. Throwable exception = this.getError(requestAttributes); if (exception instanceof MyException) { errorAttributes.put("code", ((MyException) exception).getCode()); } return errorAttributes; } }; } }
If a controller method throws MyException the response now looks like this:
{ "code": "12345", "error": "Not Found", "exception": "com.mycompany.rest.MyException", "message": "A record with value [foo] was not found", "path": "/foo", "status": 404, "timestamp": 1507117452642 }
« Back